D'ANGELO
ANGELES
CA
A simple raytracer built in C++ that renders 3D scenes in real time directly to the terminal using ASCII art. This project highlights the theoretical concepts of ray tracing, including ray-object intersection, shading, and lighting. The raytracer supports loading scenes from text files, rendering meshes, spheres, and planes. The program accelerates ray-object intersection using bounding volume hierarchies (BVH) and CPU multithreading. I hope to accelerate the raytracer further using CUDA.
This web application uses L-systems to generate and render 3D structures. An L-system is a parallel rewriting system that can be used to model the growth processes of plants and other fractal-like structures. The L-system is defined by a set of production rules that specify how to replace symbols in a string with other symbols. The system uses special control symbols in the production to control how the structure is rendered in 3D space.
I was inspired by this 3blue1brown video to use math to make stunning visuals with a surprisingly simple function ƒout(t)=∑cne-2πit, where the coefficients cn are computed from the input function cn=∫01ƒin(t)e-n2πit.
The Fourier Series is a way to represent any periodic function as a sum of sines and cosines. It can also approximate non-periodic functions as well, allowing the user to draw their own picture to approximate.
The Neural Network is a very important concept in Machine Learning and Artificial Intelligence. I wanted to dive deep into designing one to fully understand how a neural network learns with gradient descent, how forward and backward propagation work, and the interesting optimization problems of training, such as dynamically setting the learning rate with rmsprop.
My implementation is very customizable, with options to do stochastic or batch gradient descent, applying the softMax function to the output layer, and allowing the user to define their own activation functions. With this I was able to achieve a 96% accuracy on the MNIST and Iris datasets.
The online demo visualizes the trained network for the MNIST dataset, allowing the user to draw numbers to predict.
This project is a C++ implementation of a regular expression engine. I wanted to explore basic parsing techniques and study state machines. The engine supports the basic regex syntax shown in the first image, and extended features such as escape symbols, wildcards, character classes, range operators, and more. The NFA construction is modified from Thompson's Construction which reduces the size NFA to a single state per character/symbol. The second image shows an example of the constructed NFA with reduced states, while the third shows a more textbook NFA construction.
I have lots of other projects on my GitHub. Some of my other fun web projects include an LL(1) Parser Generator, an LR/LALR(1) Parser Generator, a Hash Map Visualizer, and of course the Particle Simulation that is the background of this page.
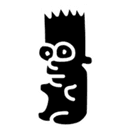
You made it all the way to the end :)
Did you like the website? Let me know, my email is in the menu at the top right.
Click to go back to the top.